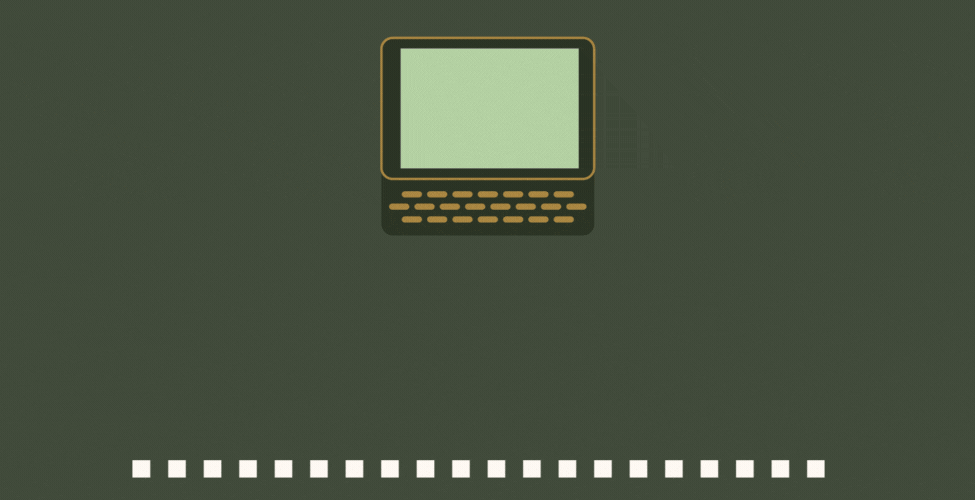
Low Code to All Code - WTH is a Variable?!
Salesforce Coding Introduction for Citizen Developers
(1) WTH is a Variable?
Variable is a way to store data in coding. There are different types of variables to hold different types of data. These types can be primitive; like text, boolean, number or it can be more complex to hold more than one value of a type like lists, sets and maps.
If you’ve used flows before, you already know what a variable is, even if you didn’t notice that you do. Let’s take a look at the below video to see how we can define a variable in flow vs in Apex.
As you may have noticed already there are 2 things defining a variable; its type and its name. A variable does not have to hold any value, even though that is the main reason we define them, but they HAVE TO have a type and a name.
Since we’ve seen how to write primitive variables, let’s take a look at more complex ones. Citizen developers probably already know how to use this first collection; lists!
One difference between defining a list in flow vs Apex is initializing the list in Apex. In Flows this is done by flow itself so you don’t have to but as a developer you’ll need to do the hard work by initializing your own list. Let’s see how to define a list in flow vs in Apex.
There are two other types of collections in Apex that are not, yet, available in Flows. These are sets and maps. My personal favorite collection is maps and I wish it was available in Flows. Fingers crossed that it will be introduced in the future.
Sets are just like lists with one big difference. They do not hold duplicated data. (The world would be a great place if our data was not duplicated but here we are.) You can define them and use them almost exactly like lists but you need to know that if you try to add the same value twice, you will still have one of it in your set.
Set<String> mascots = new Set<String>();
mascots.add('Astro');
mascots.add('Codey');
System.debug(mascots); // [Astro, Codey]
mascots.add('Astro');
System.debug(mascots); // [Astro, Codey]
Now it is time for maps. As I mentioned earlier it is my personal favorite because it allows very flexible and elegant coding, however first time coders have a little struggle in the beginning while understanding the map mechanism. If you don’t get it right away, don’t worry. It may take many tries and hours to grasp how it works but after you get it, you’ll be happier while coding.
Maps are a love child of Sets and Lists. It holds a key that gives you a value. The keys in the map need to be unique, like sets, but values can hold duplicates like lists.
Let’s see how to define maps.
Map<String, Decimal> productPrices = new Map<String, Decimal>();
productPrices.put('Bread', 1.5);
productPrices.put('Jam', 2.5);
productPrices.put('Peanut Butter', 2.5);
System.debug(productPrices); // {Bread=1.5, Jam=2.5, Peanut Butter=2.5}
We see how to define variables in Apex and how you can do the same in Flows (when available). Let me know if you have any questions or if you want to see something else in this series.